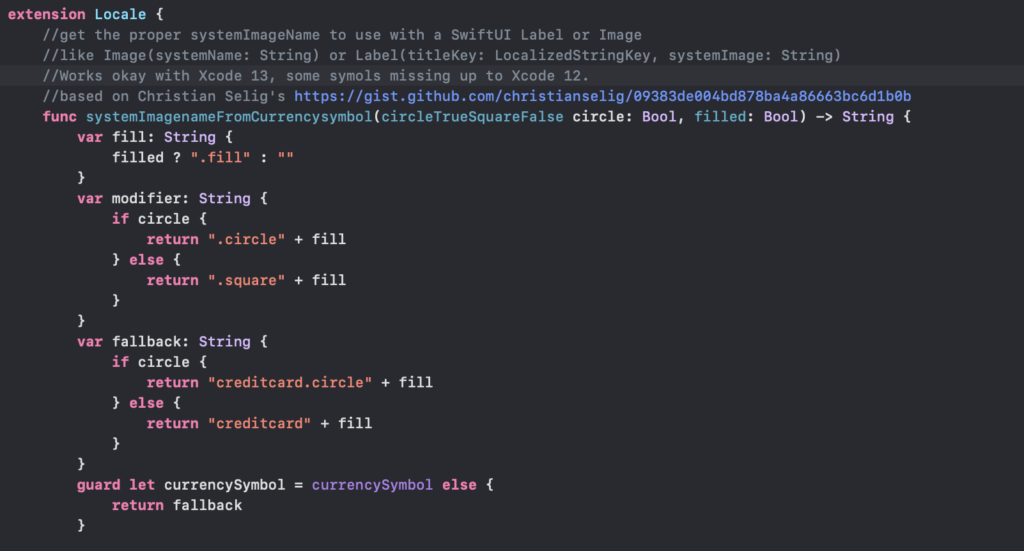
I wanted to adapt Christian Selig’s Locale+SFSymbol.swift to just give me the proper systemName of the SF Symbol to use with a SwiftUI Image or Label, like
Image(systemName: Locale.systemImagenameFromCurrencysymbol)
So here’s what I came up with:
extension Locale {
//get the proper systemImageName to use with a SwiftUI Label or Image
//like Image(systemName: String) or Label(titleKey: LocalizedStringKey, systemImage: String)
//Works okay with Xcode 13, some symols missing up to Xcode 12.
//based on Christian Selig's https://gist.github.com/christianselig/09383de004bd878ba4a86663bc6d1b0b
func systemImagenameFromCurrencysymbol(circleTrueSquareFalse circle: Bool, filled: Bool) -> String {
var fill: String {
filled ? ".fill" : ""
}
var modifier: String {
if circle {
return ".circle" + fill
} else {
return ".square" + fill
}
}
var fallback: String {
if circle {
return "creditcard.circle" + fill
} else {
return "creditcard" + fill
}
}
guard let currencySymbol = currencySymbol else {
return fallback
}
let symbols: [String: String] = [
"$": "dollar",
"¢": "cent",
"¥": "yen",
"£": "sterling",
"₣": "franc",
"ƒ": "florin",
"₺": "turkishlira",
"₽": "ruble",
"€": "euro",
"₫": "dong",
"₹": "indianrupee",
"₸": "tenge",
"₧": "peseta",
"₱": "peso",
"₭": "kip",
"₩": "won",
"₤": "lira",
"₳": "austral",
"₴": "hryvnia",
"₦": "naira",
"₲": "guarani",
"₡": "coloncurrency",
"₵": "cedi",
"₢": "cruzeiro",
"₮": "tugrik",
"₥": "mill",
"₪": "shekel",
"₼": "manat",
"₨": "rupee",
"฿": "baht",
"₾": "lari",
"R$":" brazilianreal"
]
guard let currencySymbolName = symbols[currencySymbol] else {
print(currencySymbol, "Not in the list. Bummer.")
return fallback
}
return "\(currencySymbolName)sign\(modifier)"
}
static func systemImagenameFromCurrencysymbol(circleTrueSquareFalse circle: Bool, filled: Bool) -> String {
//Shortcut for current Locale
Locale.current.systemImagenameFromCurrencysymbol(circleTrueSquareFalse: circle, filled: filled)
}
static var systemImagenameFromCurrencysymbol: String {
//convenience
Locale.current.systemImagenameFromCurrencysymbol(circleTrueSquareFalse: false, filled: false)
}
}
Note: requires Xcode 13 to (mostly) work properly. In Xcode 12 some locales are not returning the expected currency symbol but the three letter ISO code. Like RUB instead of ₽. Some currencies like CHF do not return a proper symbol even in Xcode 13. Are they using the franc symbol ₣ in Switzerland? I don’t know. So it’s probably a good idea to include a fallback image. I chose creditcard.